Rolling 3D dice with simulated physics in unity.
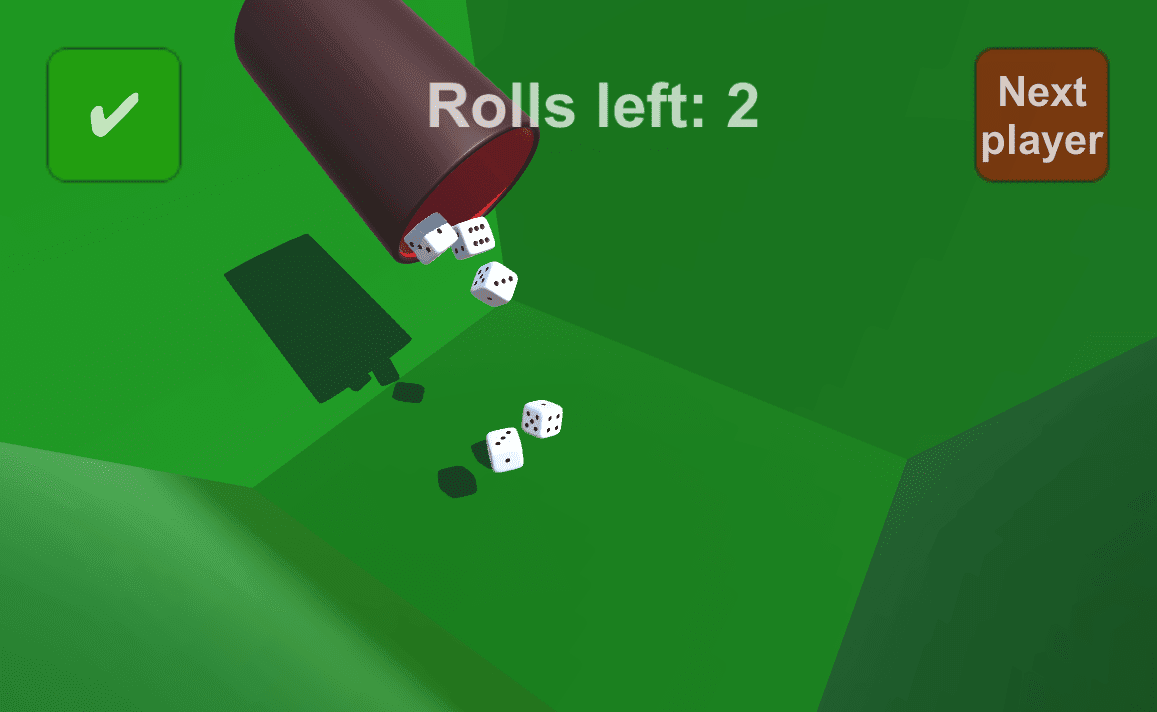
You have probably already tried at least a dozen virtual dice games right? Whether it have been simulated casino, virtual dice roller apps, or maybe a mini game in a PC or console game. I bet you that all of them have one thing in common. They are FAKE!
disclaimer: This is not a step by step tutorial, but covers some higher level considerations when making a dice roll game.
Yes, usually virtual dice games doesn't actually roll the dice. Most likely a simple random number generator will choose a number between 1 and 6, followed by an animation that will end up showing the corresponding dice face to the number the random generator picked. To be fair here, there is absolutely nothing wrong about simulating dice rolls that way. It doesn't change the players chances in the game and the rolls are still unpredictable. Or to be precise - unpredictable unless you know "the seed" to the random generator and are able to read the memory of the program. But let's just consider it unpredictable for the sake of this article.
For our simple Yahtzee score sheet app we wanted a dice roll that felt a little more realistic than the ones we have tried. First we designed a 3d dice in Blender, then a cup and imported both into Unity. Unity ships with a physics engine so you don't have to code your own from scratch, which comes in quite handy when you want to simulate physics for 3d objects. We gave the dice a rigidbody, a box collider and a material. We then tuned the friction and bounciness of the material until we were satisfied with the behavior of the dice when dropped onto a surface within unity. At this point we already have something that looks quite nice, except.... the dices makes the exact same roll each time and that totally strips away the realistic feeling.
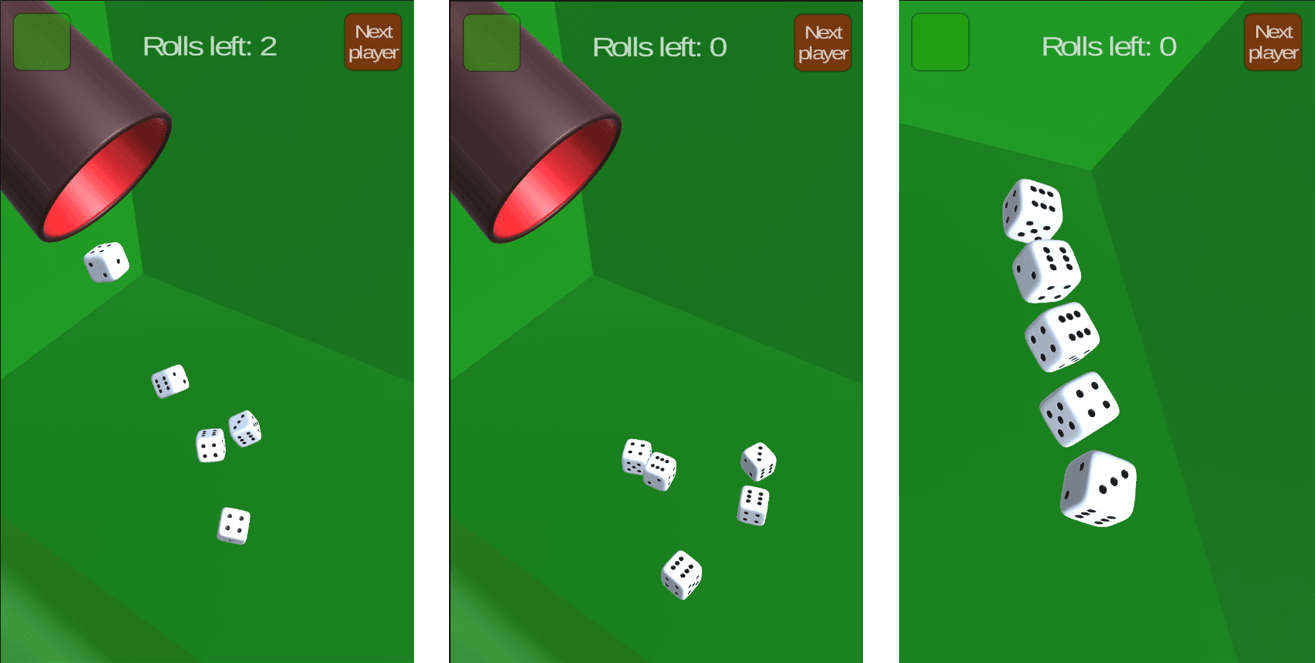
So how do we combine randomness, with physics that always behave the same?
To be straightforward honest... We used a random number generator :) But we are not choosing a random number between 1 and 6. How would that even go into the physics sequence? There would be a total 6 different rolls? That would be very boring as even the slowest mind would realize the repeating animation within a few games. Instead we decided to take advantage of the three dimensions x, y, z. Each dice is placed inside the cup where they are not visible to the player. The random generator then picks a number between 1 and 360 for each of the three axis and rotate each dice accordingly.
public void putInCup(){
if (firstRoll) {
cupPosition = gameObject.transform.position;
firstRoll = false;
}
if (!locked) {
gameObject.transform.position = cupPosition;
gameObject.transform.Rotate (
new Vector3 (
Random.Range(0f, 360f),
Random.Range(0f, 360f),
Random.Range(0f, 360f)
)
);
gameObject.SetActive (false);
}
}
That gives us an almost infinite amount of positions the dices can be places, as the number between 1 and 360 is a decimal number (float) and there are 5 dice. What is the chance that 5 (dice) ⨯ 3 (dimensions) ⨯ 360 (degrees) ⨯ 10,000,000 (7 decimals) will ever roll the same? That leaves us with a total of 54,000,000,000 different rolls that will each land on one of 7776 different possible combinations.
Pros and cons of using simulated physics to roll a dice
So you might be thinking, was it worth all the trouble? What are the drawbacks? It might seem like a lot of work building a dice rolling game in 3d with a physics engine with gravity, bounciness, friction etc. But when you think about it, the only code to write is; resetting the dice position, calculation 3 random number (one for each axis), activating the dices so that the physics apply, in this case gravity will pull them down towards the plane surface and your roll is complete. You even get the animation for free instead of writing scripts that will animate images of dice faces.
So what are the possible drawbacks? Well... first of all, since this is very random and there are so many different rolls that we don't have control over, we do sometimes end up with dices in an awkward position. I would estimate that about 1 in 30 rolls one of the dices will land on top of, or lean against another dice making that roll invalid. But this is no different from playing with dices in real life! Another possible drawback are the chances. We know how many total rolls there are based on the previous calculation, we also know how many possible combinations 5 dice can roll. We don't however, know if all of our 54 billion rolls are equally spread on the 7776 resulting combinations. If a very dedicated person would do statistics and collect enough data to prove that some combinations would occur more than other, they could use this information to gain advantage over other players. But again, that (kind of) also exist with real dices, some of them will have unequal distributed weight and will land on one side more often which could also be proven with statistics and then used to play strategical, which is what I'm gonna base my conclusion on.
Rolling virtual dices with simulated physics is FUN! it not only looks awesome but also have a way more realistic feeling to it, and most important it is very easy to build. If you ever consider to make your own dice rolling game we will be happy to discuss any questions you might have.