Unity "blink pulse" sprite animation
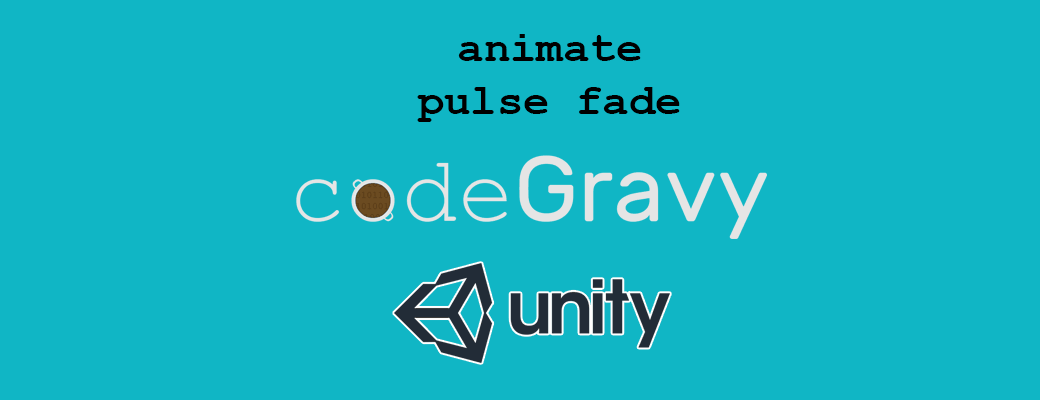
This article will teach the perhaps most easy way of fading a sprite image alpha in a pulsating pattern.
In my script folder I keep a folder called "micro", this folder contains a lot of super tiny scripts like this one. It is very easy to just attach these small scripts to your GameObjects and adjust the parameters to your need.
public class blinkPulse : MonoBehaviour {
private SpriteRenderer sr;
public float minimum = 0.3f;
public float maximum = 1f;
public float cyclesPerSecond = 2.0f;
private float a;
private bool increasing = true;
Color color;
void Start() {
sr = gameObject.GetComponent<SpriteRenderer>();
color = sr.color;
a = maximum;
}
void Update() {
float t = Time.deltaTime;
if (a >= maximum) increasing = false;
if (a <= minimum) increasing = true;
a = increasing ? a += t * cyclesPerSecond * 2 : a -= t * cyclesPerSecond;
color.a = a;
sr.color = color;
}
}
There is not a lot to this code that you need to understand. There are three public float variables, these are the only adjustable parameters you need.
- minimum: this value will be the "minimum" alpha (0.3 = 30% opacity).
- maximum: this value is the maximum opacity, most likely you want this value to be 1 (100% opacity).
- cyclesPerSecon: pretty self explanatory, this is the speed of the pulsing animation the higher the number, the higher the animation speed.
How to use
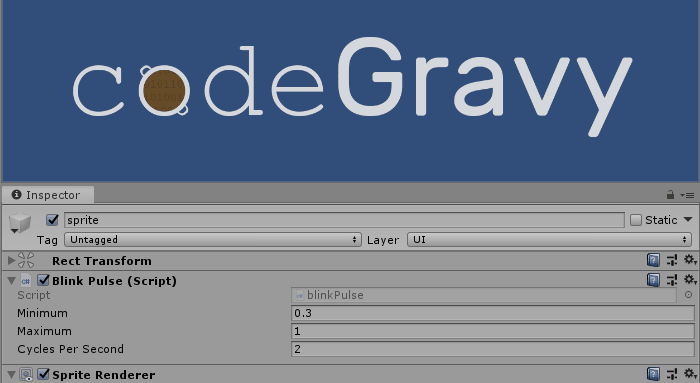
Attach the script to your sprite GameObject, and you are good to go.